Network communication is trivial in Cordova based projects. You have two main options to communicate with a server: HTTP or WebSockets.
You start having problems when you want to use something more low level. For example, if you want to implement a client to communicate with legacy services (like Telnet, IRC, etc), you need to use native TCP sockets.
Current W3C web APIs don’t expose any standardized interface and proposals like TCPSocket (https://developer.mozilla.org/en-US/docs/Web/API/TCPSocket) are still far from being implemented in web browsers.
We wanted to implement a phone and tablet application for online chess playing, that runs on three major operating systems: iOS, Android, and Windows Phone. We didn’t want to reinvent the wheel, by implementing our own chess server, so we decided to build the application on the time-honored Free Internet Chess Server (http://www.freechess.org/).
The problem is that FICS uses old fashioned TCP text based protocol, so the use of standard Web API was not an option. We could use websocket-to-socket bridge with intermediate service, but it requires hosting of service on some infrastructure, and it slightly worse reliability. Finally, we decided to implement our own Cordova plugin for TCP communication. I’ll describe it here in this blog post.
Usage
You can install plugin to your Cordova project with command line:
cordova plugin add cz.blocshop.socketsforcordova
Usage is simple, you just have to create instance of socket object:
var socket = new Socket();
Then you need to setup data consumer, error and close handlers:
socket.onData = function(data) { // invoked after new batch of data is received (typed array of bytes Uint8Array) }; socket.onError = function(errorMessage) { // invoked after error occurs during connection }; socket.onClose = function(hasError) { // invoked after connection close };
Finally you can connect to server:
socket.open( "someremoteserver.com", 1234, function() { // invoked after successful opening of socket }, function(errorMessage) { // invoked after unsuccessful opening of socket });
At this point, socket instance is in connected state and you started to receive events to handlers you setup above.
Write method of the socket accepts only Uint8Array, so you have to convert your string data. This code shows how to do it:
var dataString = "Hello world"; var data = new Uint8Array(dataString.length); for (var i = 0; i
There are 2 options of how to close the connection.
1) You can close the connection gracefully by sending FIN packet using shutdownWrite method:
socket.shutdownWrite();
After you call this method, you should wait until server sends all data to you and closes the connection. After that, you receive socket.onClose event.
2) Second options is to close socket immediately:
socket.close();
You receive socket.onClose event shortly after you call this method. Writing data to closed socket is not allowed.
More information
We created a sample Cordova project on GitHub:
https://github.com/blocshop/sockets-for-cordova-tester
The Cordova plugin itself is also available on GitHub:
https://github.com/blocshop/sockets-for-cordova
To see how this plugin works in a real-world application, you can download our Blindfold Chess Trainer game in your mobile app store (Android, iOS) and play online. Enjoy!
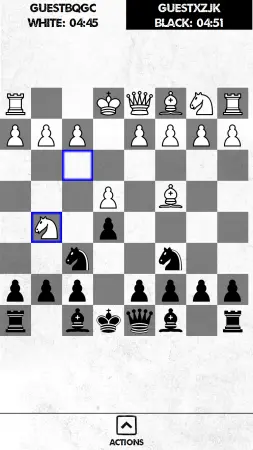